백엔드 설치 과정
1️⃣ 가장 먼저 트위터 백엔드 파일을 생성해주었다 ⇒ My-Nwitter-Backend
2️⃣ 그 다음 Node.js express 설치
→ 팀 프로젝트할 때 정리해두었던 문서를 참고하면서 설치했다
(Node.js + Express) + Typescript + ESLint 설치과정
3️⃣ Sequelize 설치
npm install --save cors dotenv mysql2 sequelize sequelize-cli reflect-metadata
npm install --save-dev @types/cors @types/validator
dotenv : Node.js 포트, DB 관련 정보 등 다양한 정보를 .env파일로 관리할 수 있게 해주는 Node.js 패키지
⇒ github에 공유가 되어서는 안되는 정보들을 .env파일에 저장해서 기존의 기능을 유지하면서 정보들을 공유하지 않도록 할 수 있다.
validator: 유효성 검사기
mysql2 : mysql과 차이점은 promise 사용여부 차이. promise를 지원하기 때문에 다른 모듈을 설치하지 않고 사용이 가능
sequelize-cli : 명령어를 사용해 데이터베이스 작업을 할 수 있는 툴.
reflect-metadata :
백엔드 실행과정에서 발생할 수 있는 문제 - CORS
cors : 도메인 또는 포트가 다른 서버의 자원을 요청하는 매커니즘
⇒ react 실행 포트와 서버 실행 포트가 다르면 CORS 오류가 발생할 수가 있다.
⇒ 이러한 오류를 해결하기 위해서 node.js 미들웨어 CORS를 추가해야함.
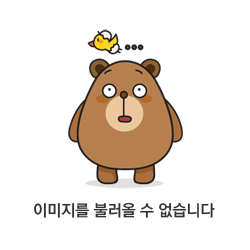
Sequelize 설치 과정
npx sequelize-cli lnit
⇒ 기본 구조 및 기본 파일 생성 (config, models, migration, seeders 폴더 생성)
config 폴더 - config.ts(DB 정보 바꾸기)
import dotenv from "dotenv";
dotenv.config();
export const config = {
development: {
username: process.env.DB_USERNAME,
password: process.env.DB_PASSWORD,
database: process.env.DB_DBNAME,
host: process.env.DB_HOST,
port: process.env.DB_PORT,
dialect: "mysql",
},
};
dotenv를 이용해서 .env파일에 데이터베이스 정보 설정.
model 생성
model 파일 - index.ts(DB와 연결할 Sequelize 객체 만들어주기)
import { Sequelize } from "sequelize-typescript";
import { config } from "../config/config";
import { Tweets } from "./tweets";
const sequelize = new Sequelize({
dialect: "mysql",
host: config.development.host,
username: config.development.username,
password: config.development.password,
database: config.development.database,
logging: false,
models: [Tweets],
});
export default sequelize;
Sequelize 객체가 DB와 잘 연결되었는지 확인 - app.ts
import express, { Request, Response, NextFunction } from "express";
import sequelize from "../models";
const app = express();
app.get("/", (req: Request, res: Response, next: NextFunction) => {
res.send("welcome!");
});
app.listen("1234", async () => {
console.log(`
################################################
🛡️ Server listening on port: 1234🛡️
################################################
`);
try {
await sequelize.authenticate();
console.log('connection success');
} catch (error) {
console.error('Unable to connect to the database:', error);
}
});
제대로 연결된거 확인.
Tweets 모델 추가 - Model 폴더 안 tweets.ts
import { Table, Column, Model, DataType } from "sequelize-typescript";
import { IntegerDataType } from "sequelize/types";
@Table({
timestamps: false,
tableName: "tweets",
charset: "utf8",
collate: "utf8_general_ci",
})
export class Tweets extends Model {
//extends 키워드는 클래스의 다른 클래스의 자식으로 만들기위해 사용.
@Column({
type: DataType.INTEGER,
autoIncrement: true,
primaryKey: true,
})
number!: IntegerDataType;
@Column({
type: DataType.STRING,
allowNull: false,
})
content!: string;
@Column({
type: DataType.STRING,
allowNull: false,
})
write_date!: string;
}
실행했을때 만든 model이 데이터베이스에 자동으로 추가되기 위해서 app.ts에 코드 추가⇒ sync() 사용
await sequelize
.sync()
.then(() => {
console.log("connection success");
})
.catch((e) => {
console.log("error: ", e);
});
'sns 프로젝트' 카테고리의 다른 글
token 검증 실패시 에러처리하기(토큰검증 미들웨어) (1) | 2024.01.21 |
---|---|
회원가입, 로그인 기능 구현(JWT) (1) | 2024.01.21 |
개인 sns 프로젝트 'NWITTER' 소개 (0) | 2024.01.21 |
재사용 컴포넌트 분리하기 (0) | 2024.01.20 |
Nwitter 프로젝트 전체 진행과정 (0) | 2024.01.20 |